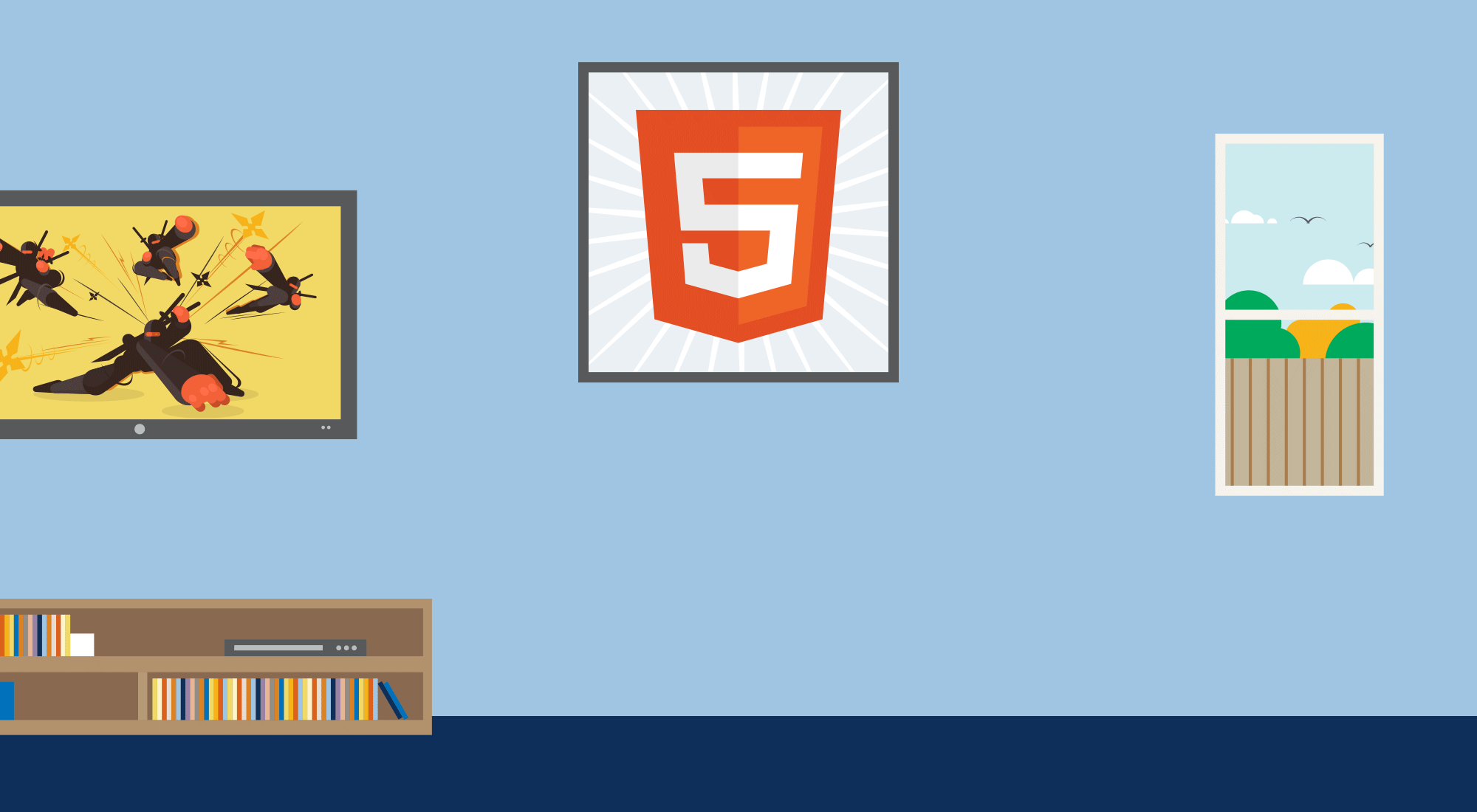
HTML5 is the latest evolution of the HTML standard. It is bundled with a lot of new elements and attributes that makes semantics, connectivity, performance, device access, 2D/3D graphics, animation and styling better on the web.
With HTML5, animations can now be programmed in the browser. Users get to enjoy all sorts of animations powered by HTML5, CSS3 and JavaScript. Apart from providing elements, such as the video and audio tag, the canvas element is a part of HTML5 that enables building games and powerful animations.
Before we dive into how the HTML5 canvas element enables us to produce several types of animations, let’s take a look at how companion technologies -- CSS3 and JavaScript -- makes animations possible with some examples on the browser.
CSS3 Animation
CSS3 ships with the transformation
and animation
properties. The transition property tells the browser to compute intermediate frames between two states. There are about eight animation properties namely:
animation-name
,animation-duration
,animation-timing-function
,animation-delay
,animation-iteration-count
,animation-direction
,animation-fill-mode
,animation-play-state
.
To make the animations play, the animation-duration property must always be specified.
In addition, it ships with keyframes
, which is the foundation of CSS animations. Keyframes actually define what the animation looks like at each stage of its timeline. Keyframes and animation go hand in hand. Check out this example.
div { -webkit-animation-duration: 2s; animation-duration: 2s; -webkit-animation-name: SkrrrPaPaPum; animation-name: SkrrrPapaPum; }
The animation name is SkrrrPapaPum
, but then we define how the animation should look like with keyframes like so:
@keyframes SkrrrPapaPum {
0% {
transform: scale(0.1);
opacity: 0;
}
60% {
transform: scale(1.2);
opacity: 1;
}
100% {
transform: scale(1);
}
}
You have the freedom to define your own style of animation using whatever properties within the keyframes scope.
Let’s take a look at some cool CSS3 animation examples.
Rain The only HTML5 element used is the
<section>
element. Peek through the CSS.html{height:100%;} body { background:#0D343A; background:-webkit-gradient(linear,0% 0%,0% 100%, from(rgba(13,52,58,1) ), to(#000000) ); background: -moz-linear-gradient(top, rgba(13,52,58,1) 0%, rgba(0,0,0,1) 100%); overflow:hidden;} .drop { background:-webkit-gradient(linear,0% 0%,0% 100%, from(rgba(13,52,58,1) ), to(rgba(255,255,255,0.6)) ); background: -moz-linear-gradient(top, rgba(13,52,58,1) 0%, rgba(255,255,255,.6) 100%); width:1px; height:89px; position: absolute; bottom:200px; -webkit-animation: fall .63s linear infinite; -moz-animation: fall .63s linear infinite; } /* animate the drops*/ @-webkit-keyframes fall { to {margin-top:900px;} } @-moz-keyframes fall { to {margin-top:900px;} }
The raindrops are gradient forms of drops that are animated to fall within a certain duration, and the directions are specified using the
keyframes
property.See the Pen CSS RAIN by raichu26 (@alemesre) on CodePen.
The JavaScript has shown in the codepen above in collaboration of the CSS properties enable the creation of raindrops.
Packman
This Packman example by Kseso involves no JavaScript at all. Just plain HTML5 and CSS3. However, the example makes heavy use of the
animation
andkeyframes
properties to make a great animation example.See the Pen Packman by Kseso by Kseso (@Kseso) on CodePen.
Twisty
We are all quite familiar with the twisty animations. These are cool because after focusing your eyes on them you can see the same movement when looking away. In this particular twisty example, the CSS3 animation property has been put to work.
html { background: black; height: 100%; position: relative; overflow: hidden; } .container{ height: 300px; width: 300px; position: absolute; top: 50%; left: 50%; margin: -150px 0 0 -150px; } .square{ height: 94%; width: 94%; background: white; position: absolute; top: 50%; left: 50%; margin: -47% 0 0 -47%; } .black{ background: black; animation: rotate 10s infinite linear; } @keyframes rotate{ 0%{ transform: rotate(0deg); } 100%{ transform: rotate(360deg); } }
The animation
and @keyframes
property are the key players in this example. The square is made to rotate 360 degrees and the duration of the animation specified to be 10 seconds, and it continually repeats itself.
See the Pen Twisty by Mike King (@micjamking) on CodePen.
HTML5 Canvas Animations
The HTML5 Canvas element is used to draw graphics on the screen with the aid of JavaScript. A simple HTML5 canvas markup can be like so:
<canvas id="myCanvas" width="200" height="100"></canvas>
And then you must use JavaScript to draw any type of shape and animations you want to achieve. A simple shape can be drawn on the canvas like so:
var c = document.getElementById("myCanvas"); var ctx = c.getContext("2d"); ctx.moveTo(0,0); ctx.lineTo(200,100); ctx.stroke();
The animation model of our solar system crafted by the guys at MDN can be shown below:
See the Pen An animated solar system by Prosper Otemuyiwa (@unicodeveloper) on CodePen.
Now, let’s take a look at some cool and mind-blowing HTML5 Canvas animations.
Canvas Waterfall
See the Pen Canvas Waterfall by Jack Rugile (@jackrugile) on CodePen.
Take a look at the JavaScript code in the codepen example above. There are a lot of computations going on. From checking if a canvas element exists to getting the 2D animation context to defining the logic for the transition of the waterfall particles to using the browser
requestAnimationFrame
andcancelAnimationFrame
API.The requestAnimationFrame method provides a smoother and more efficient way for animating by calling the animation frame when the system is ready to paint the frame. The number of callbacks is usually 60 times per second and may be reduced to a lower rate when running in background tabs.
Therefore, when creating animations, use this method instead of
setTimeout
orsetInterval
for visual updates. Thewindow.requestAnimationFrame()
method tells the browser that you wish to perform an animation and requests that the browser call a specified function to update an animation before the next repaint. The method takes an argument as a callback to be invoked before the repaint.Paul Irish wrote extensively about requestAnimationFrame . If the page is not visible to the user, requestAnimationFrame will make the animations stop.
Check out another animation demo with requestAnimationFrame.
Tearable Cloth
I’m still in awe of this tearable cloth animation demo that was created with HTML5 Canvas and JavaScript. The demo allows a user to right-click and drag their mouse to tear the cloth. Check it out below:
See the Pen Tearable Cloth by dissimulate (@dissimulate) on CodePen.
Note: The requestAnimationFrame method I mentioned earlier was used here.
Particles
This demo reflects several multi-colored bubbling particles. The animation was created on the canvas element.
See the Pen Particles by Elton Kamami (@eltonkamami) on CodePen.
Real World Lightning Effect
The power of HTML5 Canvas and JavaScript in producing real world lightning effect animation. Check out the source code. Very easy to understand.
See the Pen Canvas Lightning WIP by Jack Rugile (@jackrugile) on CodePen.
SVG Animations
The HTML5 <svg> element is a container for SVG graphics. SVG is XML-based and you can attach JavaScript event handlers to elements within the <svg> element. All modern browsers support rendering SVG with enables you reach a majority of users online without compatibility issues.
SVG is resolution-independent and best suited for applications with large rendering areas, such as Google Maps. It is not suited for game applications. However, Canvas is resolution dependent. It has no support for event handlers and the text rendering capabilities are poor. Canvas is great for graphic-intensive games.
Become a Traveller Today
This is an amazing SVG animation created using SVG and CSS. The CSS animation properties were very useful in ensuring it is as good as it looks and moves.
See the Pen SVG Animation by jjperezaguinaga (@jjperezaguinaga) on CodePen.
Breaking Bad
Breaking Bad series fans, this is for you. A beautiful combination of SVG, Canvas, CSS and JavaScript.
See the Pen Breaking Bad by Tim Pietrusky (@TimPietrusky) on CodePen.
Rainbow Rocket Man
This is an animation of an astronaut ascending into space. SVG, CSS and JavaScript.
See the Pen SVG Rainbow Rocket Man by Chris Gannon (@chrisgannon) on CodePen.
Conclusion
Before now, Flash was heavily used to make animations on the web. Butit was expensive to develop and deploy animations to the web. Now, HTML5 makes animations easily accessible and powerful. As we saw in the examples above, you can create very brilliant animations with the help of HTML5, CSS3 and JavaScript.
Go forth and animate!